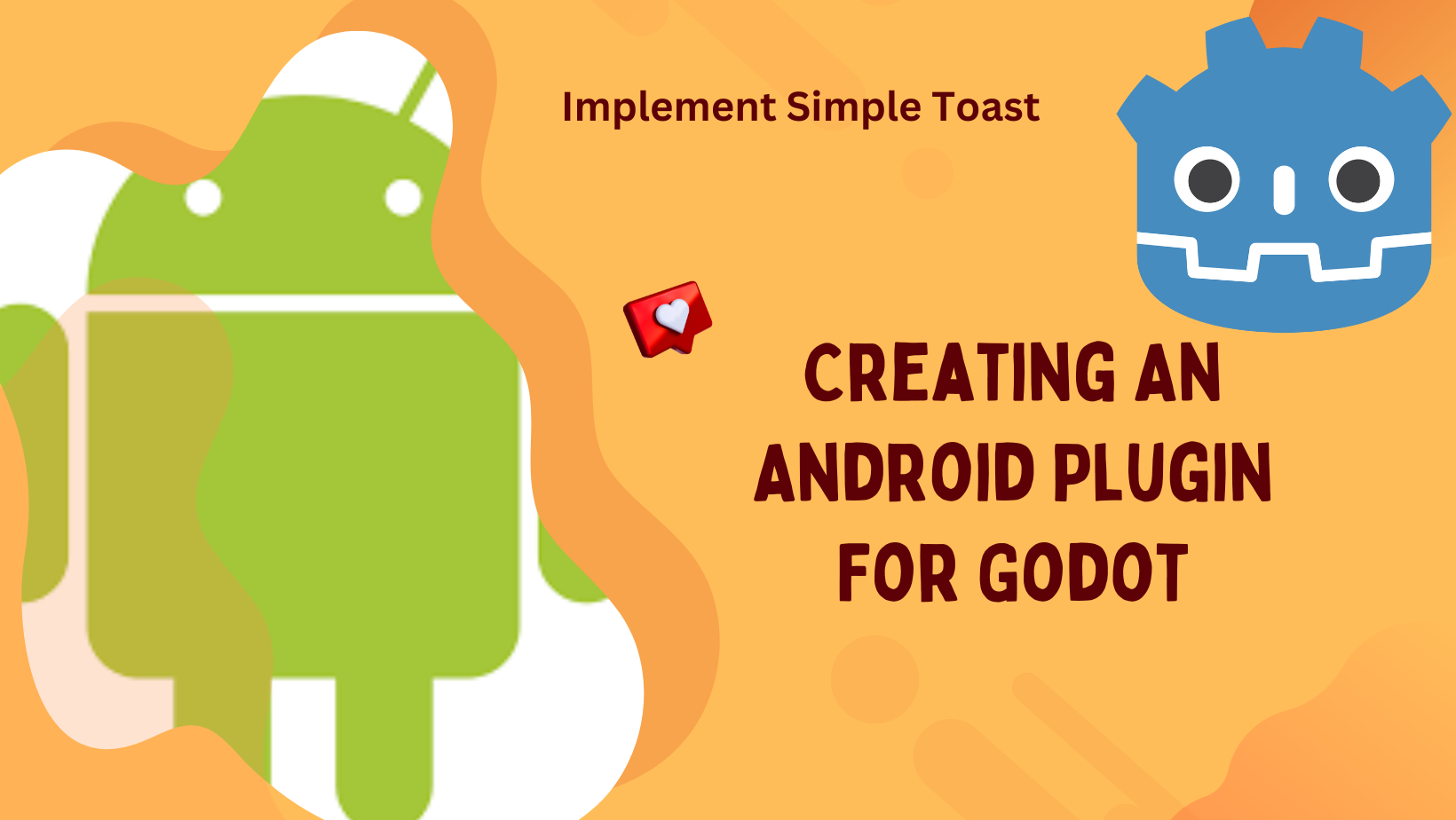
Creating an Android Plugin for Godot
Hello there, In this article I will be writing about the process to create an android plugin. Here we will implement a simple functionality of android (a Toast) and call that function through Godot.
I don't have much experience with Godot and Android, but I have published few android apps, and few Godot games. This guide is primarily for myself to document the process and reference it in future when needed. But if you were looking for some user perspective document on creating an Android plugin for Godot, here it is for you as well.
I assume that you already have android studio installed and Java environment is setup. You don't have to worry about Godot, you can use the stable release, at least for this simple demo android plugin.
Let's get started. I am following this Godot official document. Let's start a new project in Android Studio. Simply choose New Project -> No Activity -> Give some details (name, package name, path, choose language, and choose minimum SDK) -> and Click Finish.
We have to create a library module, but I can't find if we can create one without creating this project. But we can convert recently created app into library as described on this Android Developer document.
From the build.gradle (app level) remove that applicationId line. ( was 10th line ), and from the same file, look for id 'com.android.application' which is in plugins and change that to id 'com.android.library'
plugins {
id 'com.android.application'
}
android {
namespace 'com.bloggernepal.godotandroidessentials'
compileSdk 34
defaultConfig {
applicationId "com.bloggernepal.godotandroidessentials"
minSdk 19
.......
to
plugins {
id 'com.android.library'
}
android {
namespace 'com.bloggernepal.godotandroidessentials'
compileSdk 34
defaultConfig {
minSdk 19
.......
Click on Sync Now or click File > Sync Project with Gradle Files.
Since we have converted the existing app, we must remove it's reference from the project level build.gradle as well. From that file remove the id 'com.android.application'.
// Top-level build file where you can add configuration options common to all sub-projects/modules.
plugins {
id 'com.android.application' version '8.0.0' apply false
id 'com.android.library' version '8.0.0' apply false
}
to
// Top-level build file where you can add configuration options common to all sub-projects/modules.
plugins {
id 'com.android.library' version '8.0.0' apply false
}
Then Click on Sync Now or click File > Sync Project with Gradle Files.
From the Run/Debug configuration, you may choose to remove the app config as well.
Let's Download Godot Engine library for Android from Godot Download Page, scroll down to Other Godot downloads and from AAR library for Android, Download the AAR Library. AAR -> Android Archive.
I have download the file, renamed it to godot-lib.3.5.3.aar, and placed it inside app/libs of the Android Project.
and added following in the build.gradle (app level)
....
dependencies {
compileOnly fileTree(dir:"libs", include:["godot-lib.3.5.3.aar"])
....
Let's create a new class that extends from org.godotengine.godot.plugin.GodotPlugin.
package com.bloggernepal.godotandroidessentials;
import androidx.annotation.NonNull;
import org.godotengine.godot.Godot;
import org.godotengine.godot.plugin.GodotPlugin;
public class AndroidEssentials extends GodotPlugin {
public AndroidEssentials(Godot godot) {
super(godot);
}
@NonNull
@Override
public String getPluginName() {
return null;
}
}
Let's update the AndroidManifest.xml.
We added this to <application></application>.
<meta-data
android:name="org.godotengine.plugin.v1.[PluginName]"
android:value="[plugin.init.ClassFullName]" />
By replacing the placeholders.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
<application >
<meta-data
android:name="org.godotengine.plugin.v1.GodotAndroidEssentials"
android:value="com.bloggernepal.godotandroidessentials.AndroidEssentials" />
</application>
</manifest>
Let's delete the entire contents on app/src/main/res as we are not using any of those.
That's it, we completed the setup.
Let's add a simple logic of Toast.
@UsedByGodot
public void showToast(String message, String duration) {
Log.i("godot", "Showing Toast");
final int durationInt = duration.equals("short") ? Toast.LENGTH_SHORT : Toast.LENGTH_LONG;
getActivity().runOnUiThread(
new Runnable() {
@Override
public void run() {
Toast.makeText(getActivity(), message, durationInt).show();
}
});
}
Let's build the project
$ ./gradlew build
inside app/build/outputs/aar, you can find two aars. app-debug.aar and app-release.aar.
We will use the release one as plugin in out Godot Game.
Let's create a Godot Project
I have created a simple Control Node and have a Button there. We will add the functionality later. I want to show the toast when clicking the button.
Let's install Android Build Template from Project -> Install Android Build Templates.
Let's Add Android Export from Project -> Exports -> + and select Android.
Choose the scene as a main Scene, Connect your phone. (you need to enable, USB Debugging) and run the application on your phone.
Let's move that app-release.aar into godot's android/plugins folder. Its better to rename the file same as the library name so let's rename it to GodotAndroidEssentials.aar, We have to create a Godot Android Plugin configuration file to help Godot detect and load the plugin. Let's create a GodotAndroidEssentials.gdap file and place it inside android/plugins (of godot project) and have these contents.
[config]
name="GodotAndroidEssentials"
binary_type="local"
binary="GodotAndroidEssentials.aar"
Now we can use this plugin while building for android, for that go to Projects -> Export... -> select Android, now check on Use Custom Build and Godot Android Essentials under plugins.
Click in the Export, it will generate a .apk. file
It should be able to generate the .apk file, if not it will throw the errors you have to identify it.
Now Let's add a script to the Control, and check if the Library is loaded or not.
extends Control
var GodotAndroidEssentials = null
func _ready():
if(Engine.has_singleton("GodotAndroidEssentials")):
print("GodotAndroidEssentials found")
GodotAndroidEssentials = Engine.get_singleton("GodotAndroidEssentials")
else:
print("GodotAndroidEssentials Not found")
pass
If you run the project in Android with "Deploy with Remote Debug" option checked from Debug Menu. you should see that "GodotAndroidEssentials found" being printed.
I encountered a tiny type and looked for a hours. $ adb logcat -c GodotPluginRegistry will be a good friend in need.
Now the final one, let's call the showToast method from Godot.
I will connect the pressed event in the button, and call the showToast after checking that the GodotAndroidEssentials exists.
func _on_Button_pressed():
if GodotAndroidEssentials:
GodotAndroidEssentials.showToast("Hey! this message is from godot", "long")
pass # Replace with function body.
Now run the application in Android, click the button. There you go there is the Toast, and message from Godot.
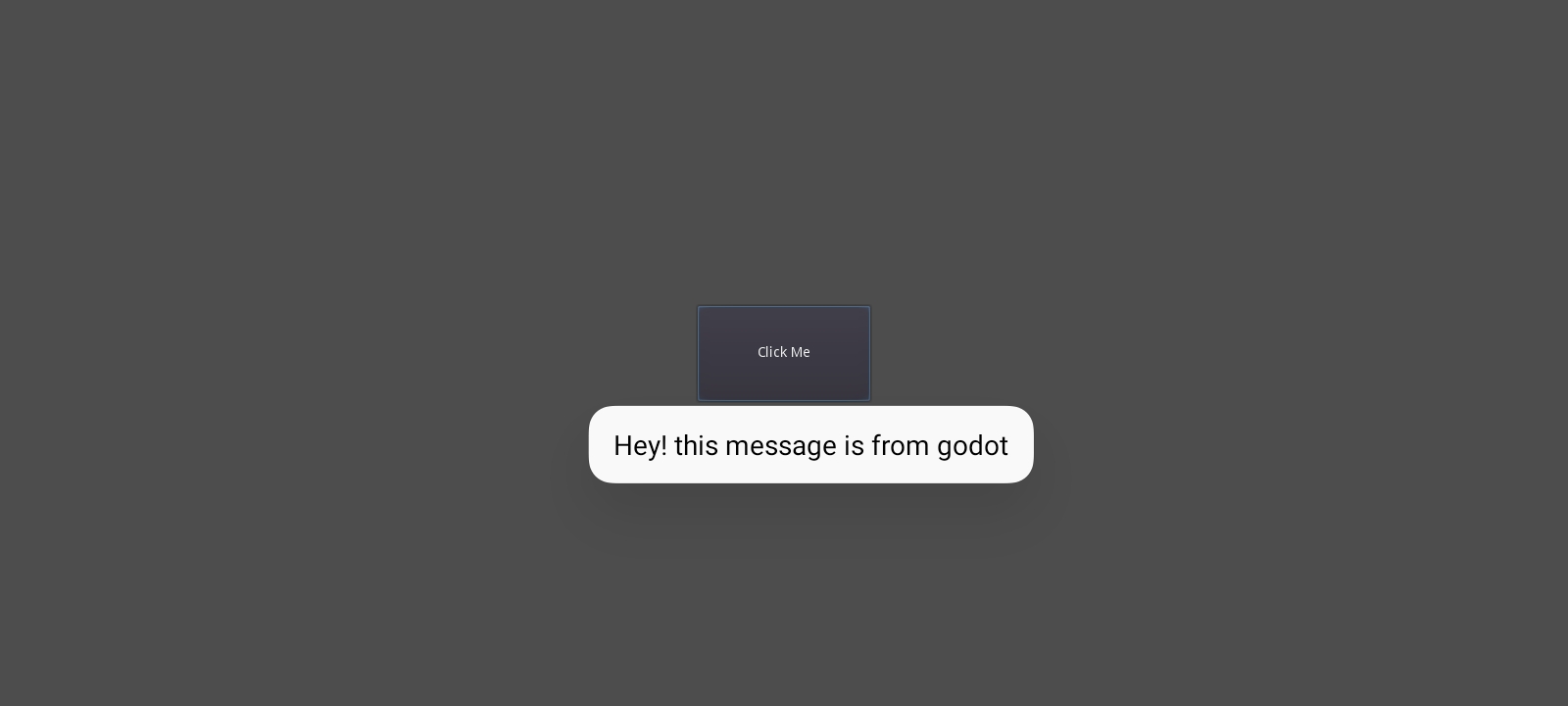
This is the simplest plugin, but you should get the gist of the plugin development process.
You can find the repository here: Creating Android Plugin.
Special thank to Shin-NiL and Randy Tan, Constantin Gisca . For creating Plugins for Admob and Play Service. I had used and gone through those plugins while creating a android plugin for Godot.
So In this article, we discussed about the process to create an Android Plugin for Godot.